Die Bibliothek u8g2 kann eine Vielzahl von OLED-Displays ansteuern.
![]() | ![]() | ![]() | |
Typbezeichnung | OLED 0,96 Zoll SSD1306 | OLED 1,3 Zoll SH1106 | OLED 2,42 Zoll SSD1309 |
Anschluss | I2C | I2C | SPI |
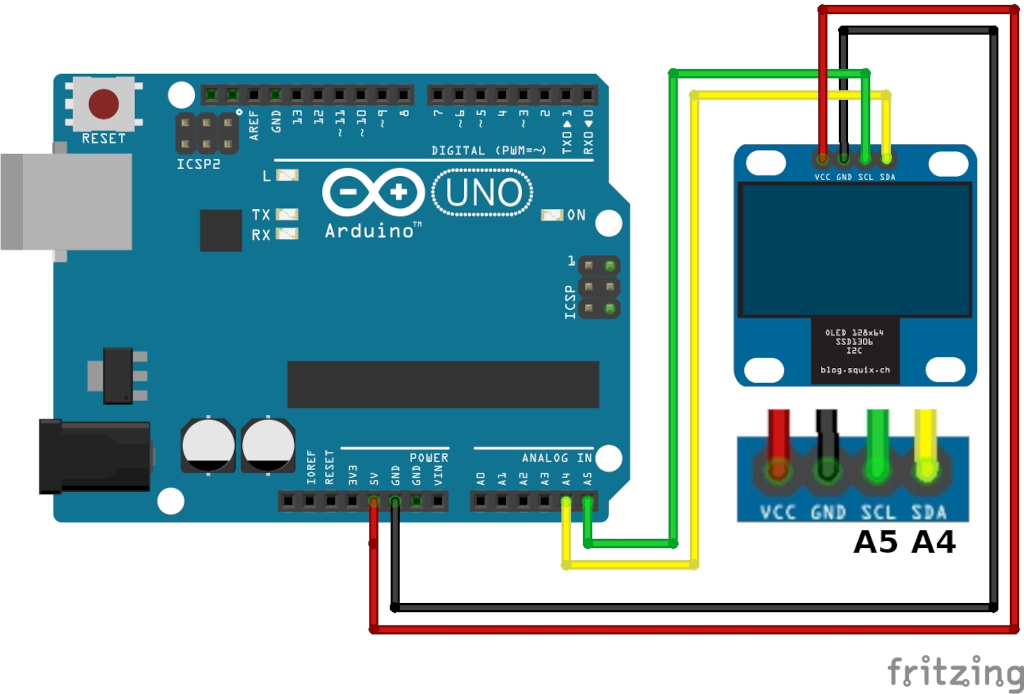
Pinbelegung eines OLED-Displays mit I2C am Arduino
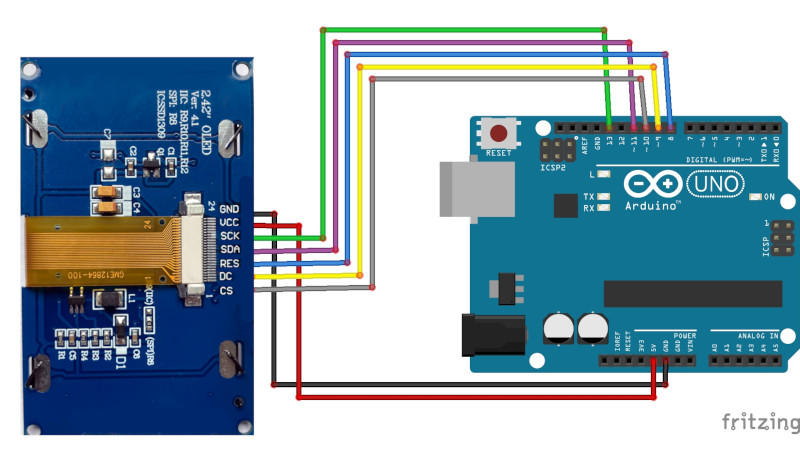
Pinbelegung eines OLED mit SPI
GND -> GND
VCC -> 5V
SCK -> 13
SDA -> 11
RS -> 8
DC -> 9
CS -> 10
Benötigte Bibliothek:
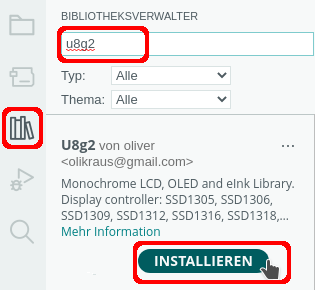
Die verschiedenen OLEDs müssen je nach Typ unterschiedlich initialisiert werden:
0,96 Zoll mit I2C-Ansteuerung (SSD1306)
U8G2_SSD1306_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
1,3 Zoll mit I2C-Ansteuerung (SH1106)
U8G2_SH1106_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
2,42 Zoll mit SPI-Ansteuerung (SSD1309)
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
Bibliothek einbinden und Display initialisieren
# include "U8g2lib.h"
// 1,3 Zoll SH1106
U8G2_SH1106_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 0,96 Zoll SSD1306
// U8G2_SSD1306_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
Ansteuerung
Hier sollen die Displays mit den Chipsätzen SSD1306 und SH1106 und I2C betrachtet werden.
Die Bibliothek kennt zwei verschiedene Modi den Bildschirm anzusprechen:
Page buffer mode: langsam, wenig Speicherbedarf
Full screen buffer mode schnell, sehr hoher Speicherbedarf mit dem Hinweis Speicherplatz- und Stabilitätsprobleme beim UNO R3
Sie unterscheiden sich in der Initialisierung und in der Programmierung:
Page buffer mode (1 hinter NONAME)
/*
Typbezeichnung mit Bildschirmgröße in Pixeln
1 = page buffer mode, F = full screen buffer mode
Hardware I2C/Hardware SPI
Name des OLEDs
Rotation R0 (keine)
*/
// 0,96 Zoll SSD1306
U8G2_SSD1306_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 1,3 Zoll SH1106
// U8G2_SH1106_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
// U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
Full screen buffer mode (F hinter NONAME)
// 0,96 Zoll SSD1306
U8G2_SSD1306_128X64_NONAME_F_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 1,3 Zoll SH1106
// U8G2_SH1106_128X64_NONAME_F_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
// U8G2_SSD1309_128X64_NONAME2_F_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
Darstellung der Inhalte
Page buffer mode:
# include "U8g2lib.h"
/*
Typbezeichnung mit Bildschirmgröße in Pixeln
1 = page buffer mode, F = full screen buffer mode
Hardware I2C/Hardware SPI
Name des OLEDs
Rotation R0 (keine)
*/
// 0,96 Zoll SSD1306
U8G2_SSD1306_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 1,3 Zoll SH1106
// U8G2_SH1106_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
// U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
void setup()
{
oled.begin();
}
void loop()
{
// Farbe weiß
oled.setDrawColor(1);
oled.firstPage();
do
{
// Rahmen mit abgerundeten Ecken an den Bildschirmrändern zeichnen
oled.drawRFrame(0, 0, 128, 64, 5);
}
while (oled.nextPage());
}
Full screen buffer mode:
# include "U8g2lib.h"
/*
Typbezeichnung mit Bildschirmgröße in Pixeln
1 = page buffer mode, F = full screen buffer mode
Hardware I2C/Hardware SPI
Name des OLEDs
Rotation R0 (keine)
*/
// 0,96 Zoll SSD1306
U8G2_SSD1306_128X64_NONAME_F_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 1,3 Zoll SH1106
// U8G2_SH1106_128X64_NONAME_F_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
// U8G2_SSD1309_128X64_NONAME2_F_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
void setup()
{
oled.begin();
}
void loop()
{
// Farbe weiß
oled.setDrawColor(1);
oled.clearBuffer();
// Rahmen mit abgerundeten Ecken an den Bildschirmrändern zeichnen
oled.drawRFrame(0, 0, 128, 64, 5);
oled.sendBuffer();
}
Funktionen der Bibliothek u8g2
Schlüsselwort | Parameter | Aktion |
---|---|---|
begin(); | OLED starten | |
getDisplayWidth(); | Bildschirmbreite feststellen | |
getDisplayHeight(); | Bildschirmhöhe feststellen | |
clearDisplay(); | Bildschirm dunkel schalten | |
setdrawColor(Parameter) | 0 → schwarz 1 → weiß | Zeichenfarbe festlegen |
setContrast(Parameter) | 0 ... 255 | Kontrast einstellen |
setDisplayRotation(U8G2_R*); | U8G2_R0 → 0 Grad U8G2_R1 → 90 Grad U8G2_R2 → 180 Grad U8G2_R3 → 270 Grad | Anzeige drehen |
flipMode(Parameter); | 0 → normale Ausrichtung 1 → 180 Grad drehen wirksam erst bei einer Rotation | Anzeige spiegeln (180 Grad) |
home(); | Cursor in die linke obere Ecke setzen | |
drawPixel(x-Achse, y-Achse) | einzelnen Pixel zeichnen | |
drawLine(StartX, StartX, EndeX, EndeY); | Linie zeichnen | |
drawHLine(StartX, StartY, Länge); | horizontale Linie zeichnen | |
drawVLine(StartX, StartY, Länge); | vertikale Linie zeichnen | |
drawFrame(StartX, StartY,, Breite, Höhe); | Rechteck zeichnen | |
drawRFrame(StartX, StartY, Breite, Höhe, Eckenradius); | abgerundetes Rechteck zeichnen | |
drawBox(StartX, StartY, Breite, Höhe); | ausgefülltes Rechteck zeichnen | |
drawCircle(MittelpunkX, MittelpunktY, Radius, Kreisausschnitt); | U8G2_DRAW_UPPER_RIGHT U8G2_DRAW_UPPER_LEFT U8G2_DRAW_LOWER_RIGHT U8G2_DRAW_LOWER_LEFT U8G2_DRAW_ALL | Kreis zeichnen Viertelkreis oben rechts Viertelkreis oben links Viertelkreis unten rechts Viertelkreis unten links voller Kreis |
drawDisc(MittelpunkX, MittelpunktY, Radius); | Ausgefüllten Kreis zeichnen | |
drawEllipse(StartX, StartY, RadiusX, RadiusY); | Ellipse zeichnen | |
drawXBM(StartX, StartY, Breite, Höhe, Array_Bilddatei); | XBM-Bild anzeigen | |
setCursor(x-Achse, y-Achse); | Cursor setzen | |
setFont(Schriftart) | Beispiele für funktionierende Schriftarten: Schrifthöhe in Pixeln (px) 6px: u8g2_font_5x7_tr 7px: u8g2_font_torussansbold8_8r 8px: u8g2_font_ncenB08_tr 10px: u8g2_font_t0_15b_me 12px: u8g2_font_helvB12_tf 13px: u8g2_font_t0_22_te 14px: u8g2_font_helvB14_tf 17px: u8g2_font_timB18_tf 18px: u8g2_font_lubB18_tr 20px: u8g2_font_courB24_tf 23px: u8g2_font_timB24_tf 25px: u8g2_font_helvR24_tf 32px: u8g2_font_logisoso32_tf 42px: u8g2_font_fub42_tf 58px: u8g2_font_logisoso58_tf 62px: u8g2_font_logisoso62_tn | Schriftart |
print("Text"); drawStr(StartX, StartY ,"Text"); | Text schreiben | |
setFontDirection(Wert); | 0 → normal ausgerichtet 1 → 90 ° gedreht 2 → 180 ° gedreht 3 → 270 ° gedreht | Schreibrichtung |
Quelle: 🔗https://github.com/olikraus/u8g2/wiki/u8g2referencel

weitere Schriftarten: 🔗https://github.com/olikraus/u8g2/wiki/fntlistall (abgerufen am 15.07.24)
Du musst ausprobieren, welche Schriftarten dargestellt werden können!
Grafik
![]() | ![]() | ![]() | ![]() |
draw(XBM(); | drawDisc(); | drawCircle(); | drawLine(); |
So sieht es auf dem 0,96 Zoll OLED aus:
So sieht es auf dem 2,42 Zoll OLED aus:

# include "U8g2lib.h"
// 0,96 Zoll SSD1306
U8G2_SSD1306_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 1,3 Zoll SH1106
// U8G2_SH1106_128X64_NONAME_1_HW_I2C oled(U8G2_R0, U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
// U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
// Bildschirmgröße
int BildschirmBreite = oled.getDisplayWidth();
int BildschirmHoehe = oled.getDisplayHeight();
// Smiley XBM erstellt mit GIMP
# define SmileyBreite 46
# define SmileyHoehe 45
static unsigned char Smiley[] =
{
0x00, 0x00, 0xfe, 0x1f, 0x00, 0x00, 0x00, 0xc0, 0xff, 0xff, 0x00, 0x00,
0x00, 0xf0, 0x07, 0xf8, 0x03, 0x00, 0x00, 0xfc, 0x00, 0xc0, 0x0f, 0x00,
0x00, 0x3e, 0x00, 0x00, 0x1f, 0x00, 0x80, 0x0f, 0x00, 0x00, 0x7c, 0x00,
0xc0, 0x07, 0x00, 0x00, 0xf8, 0x00, 0xe0, 0x01, 0x00, 0x00, 0xe0, 0x01,
0xf0, 0x00, 0x00, 0x00, 0xc0, 0x03, 0x70, 0x00, 0x00, 0x00, 0x80, 0x03,
0x38, 0x7e, 0x00, 0x80, 0x1f, 0x07, 0x38, 0xff, 0x00, 0xc0, 0x3f, 0x07,
0x9c, 0xff, 0x01, 0xc0, 0x3f, 0x0e, 0x9c, 0xe7, 0x01, 0xc0, 0x39, 0x0e,
0x8e, 0xc3, 0x01, 0xc0, 0x30, 0x1c, 0x8e, 0xe3, 0x01, 0xc0, 0x31, 0x1c,
0x86, 0xf7, 0x01, 0xc0, 0x3b, 0x18, 0x87, 0xff, 0x01, 0xc0, 0x3f, 0x38,
0x07, 0xff, 0x00, 0x80, 0x3f, 0x38, 0x03, 0x7e, 0x00, 0x80, 0x1f, 0x30,
0x03, 0x00, 0x00, 0x00, 0x00, 0x30, 0x03, 0x00, 0x00, 0x00, 0x00, 0x30,
0x03, 0x00, 0x00, 0x00, 0x00, 0x30, 0x03, 0x00, 0x00, 0x00, 0x00, 0x30,
0x03, 0x00, 0x00, 0x00, 0x00, 0x30, 0x03, 0x00, 0x00, 0x00, 0x00, 0x30,
0x07, 0x00, 0x00, 0x00, 0x00, 0x38, 0x07, 0x20, 0x00, 0x00, 0x01, 0x38,
0x06, 0x70, 0x00, 0x80, 0x03, 0x18, 0x0e, 0xf0, 0x00, 0xc0, 0x01, 0x1c,
0x0e, 0xe0, 0x03, 0xf0, 0x01, 0x1c, 0x1c, 0xc0, 0x3f, 0xfc, 0x00, 0x0e,
0x1c, 0x80, 0xff, 0x7f, 0x00, 0x0e, 0x38, 0x00, 0xfc, 0x1f, 0x00, 0x07,
0x38, 0x00, 0xc0, 0x03, 0x00, 0x07, 0x70, 0x00, 0x00, 0x00, 0x80, 0x03,
0xf0, 0x00, 0x00, 0x00, 0xc0, 0x03, 0xe0, 0x01, 0x00, 0x00, 0xe0, 0x01,
0xc0, 0x07, 0x00, 0x00, 0xf8, 0x00, 0x80, 0x0f, 0x00, 0x00, 0x7c, 0x00,
0x00, 0x3e, 0x00, 0x00, 0x1f, 0x00, 0x00, 0xfc, 0x00, 0xc0, 0x0f, 0x00,
0x00, 0xf0, 0x07, 0xf8, 0x03, 0x00, 0x00, 0xc0, 0xff, 0xff, 0x00, 0x00,
0x00, 0x00, 0xfe, 0x1f, 0x00, 0x00
};
// Schneemann XBM erstellt mit GIMP
# define SchneemannBreite 28
# define SchneemannHoehe 62
static unsigned char Schneemann[] =
{
0x00, 0xf0, 0x01, 0x00, 0x00, 0xfc, 0x07, 0x00, 0x00, 0x0e, 0x06, 0x00,
0x00, 0x06, 0x0c, 0x00, 0x00, 0x02, 0x08, 0x00, 0x00, 0x03, 0x18, 0x00,
0x00, 0x03, 0x18, 0x00, 0x00, 0x03, 0x18, 0x00, 0x00, 0x03, 0x38, 0x00,
0xe0, 0xff, 0xff, 0x03, 0x00, 0xfe, 0x0f, 0x00, 0x00, 0x0f, 0x1e, 0x00,
0x80, 0x03, 0x1c, 0x00, 0x80, 0x01, 0x38, 0x00, 0xc0, 0x19, 0x37, 0x00,
0xc0, 0x1c, 0x37, 0x00, 0xc0, 0x18, 0x27, 0x00, 0xc0, 0x00, 0x20, 0x00,
0xc0, 0x08, 0x21, 0x00, 0xc0, 0xf9, 0x31, 0x00, 0xc0, 0xf1, 0x38, 0x00,
0x80, 0x03, 0x38, 0x00, 0x80, 0x07, 0x1c, 0x00, 0x00, 0x1f, 0x0f, 0x00,
0x00, 0xfc, 0x07, 0x00, 0x00, 0xfc, 0x03, 0x04, 0x00, 0xff, 0x0f, 0x06,
0x80, 0x07, 0x1e, 0x06, 0xc0, 0x03, 0x3c, 0x03, 0xe0, 0x00, 0x70, 0x03,
0xf0, 0x00, 0xf0, 0x01, 0x70, 0x00, 0xe0, 0x00, 0x38, 0x00, 0xc0, 0x01,
0x38, 0xf0, 0xc0, 0x01, 0x18, 0xf0, 0xe0, 0x01, 0x18, 0xf0, 0xb0, 0x01,
0x0c, 0x70, 0x30, 0x03, 0x0c, 0x00, 0x18, 0x03, 0x0c, 0x00, 0x18, 0x03,
0x0e, 0x00, 0x08, 0x07, 0x06, 0x00, 0x0f, 0x06, 0x06, 0x00, 0x0f, 0x06,
0x06, 0x30, 0x06, 0x06, 0x06, 0x70, 0x00, 0x06, 0x06, 0xf0, 0x00, 0x06,
0x06, 0xf0, 0x00, 0x06, 0x0e, 0x60, 0x00, 0x07, 0x0c, 0x00, 0x00, 0x03,
0x0c, 0x00, 0x00, 0x03, 0x0c, 0x00, 0x00, 0x03, 0x0c, 0x00, 0x00, 0x03,
0x18, 0x00, 0x80, 0x01, 0x38, 0x60, 0xc0, 0x01, 0x38, 0xf0, 0xc0, 0x01,
0x78, 0xf0, 0xe0, 0x00, 0xf0, 0xf0, 0xf0, 0x00, 0xf0, 0x00, 0x70, 0x00,
0xe0, 0x03, 0x7c, 0x00, 0xe0, 0x07, 0x3e, 0x00, 0xe0, 0xff, 0x3f, 0x00,
0xc0, 0xff, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x00
};
void setup()
{
// Zufallsgenerator starten
randomSeed(A0);
// Display starten
oled.begin();
// Kontrast maximal 255
oled.setContrast(200);
}
void loop()
{
// Farbe weiß
oled.setDrawColor(1);
// Smiley
oled.firstPage();
do
{
oled.drawXBM(40, 10, SmileyBreite, SmileyHoehe, Smiley);
}
while (oled.nextPage());
delay(2000);
// Schneemann
oled.firstPage();
do
{
oled.drawXBM(50, 1, SchneemannBreite, SchneemannHoehe, Schneemann);
}
while (oled.nextPage());
delay(2000);
// Pixelmuster
oled.firstPage();
do
{
for (int i = 0; i < 500; i ++)
{
int x = random(1, BildschirmBreite);
int y = random(1, BildschirmHoehe);
oled.drawPixel(x, y);
}
}
while (oled.nextPage());
delay(2000);
oled.setFont(u8g2_font_unifont_t_symbols);
// Text horizontal
oled.firstPage();
do
{
oled.setFontDirection(0);
oled.setCursor(2, BildschirmHoehe / 2);
oled.print("Text");
}
while (oled.nextPage());
delay(2000);
// Text 90 Grad gedreht
oled.firstPage();
do
{
oled.setFontDirection(1);
oled.setCursor(BildschirmBreite / 2, 2);
oled.print("Text");
}
while (oled.nextPage());
delay(2000);
// Text 180 Grad gedreht
oled.firstPage();
do
{
oled.setFontDirection(2);
oled.setCursor(BildschirmBreite - 2, BildschirmHoehe / 2);
oled.print("Text");
}
while (oled.nextPage());
delay(2000);
// Text 270 Grad gedreht
oled.firstPage();
do
{
oled.setFontDirection(3);
oled.setCursor(BildschirmBreite / 2, BildschirmHoehe - 2);
oled.print("Text");
}
while (oled.nextPage());
delay(2000);
// Kreise
oled.firstPage();
do
{
for (int i = 2; i < BildschirmHoehe / 2; i += 3)
{
oled.drawCircle(BildschirmBreite / 2, BildschirmHoehe / 2, i);
}
}
while (oled.nextPage());
delay(2000);
// Rahmen
oled.firstPage();
do
{
for (int i = 2; i < BildschirmHoehe; i += 4)
{
oled.drawFrame(0, 0, i, i);
}
}
while (oled.nextPage());
delay(2000);
// vertikale Linie
oled.firstPage();
do
{
for (int i = 0; i < BildschirmBreite; i += 4)
{
oled.drawVLine(i, 0, BildschirmBreite - 1);
}
}
while (oled.nextPage());
delay(2000);
// horizontale Linie
oled.firstPage();
do
{
for (int i = 0; i < BildschirmHoehe; i += 4)
{
oled.drawHLine(0, i, BildschirmBreite - 1);
}
}
while (oled.nextPage());
delay(2000);
// ausgefüllte Kreise
oled.firstPage();
do
{
int Radius = 5;
int StartX = 10;
int StartY = 10;
while (StartX < BildschirmBreite - Radius)
{
for (int i = StartY; i < BildschirmBreite - Radius; i += 20)
{
oled.drawDisc(StartX, i, Radius);
}
StartX += 10;
}
}
while (oled.nextPage());
delay(2000);
// Linien
oled.firstPage();
do
{
for (int i = 0; i < BildschirmBreite; i += 5)
{
oled.drawLine(0, i, 128, 64);
}
for (int i = BildschirmBreite; i > 0; i -= 5)
{
oled.drawLine(BildschirmBreite, i, 0, 0);
}
}
while (oled.nextPage());
delay(2000);
}
Bildschirm drehen

Beispiel
# include "U8g2lib.h"
// 0,96 Zoll SSD1306
U8G2_SH1106_128X64_NONAME_1_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE);
// 1,3 Zoll SH1106
// U8G2_SSD1306_128X64_NONAME_1_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE);
// 2,42 Zoll SSD1309
// U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI oled(U8G2_R0, 10, 9, 8);
int BildschirmBreite = u8g2.getDisplayWidth();
int BildschirmHoehe = u8g2.getDisplayHeight();
// Smiley
# define SmileyBreite 46
# define SmileyHoehe 45
static unsigned char Smiley[] =
{
0x00, 0x00, 0xfe, 0x1f, 0x00, 0x00, 0x00, 0xc0, 0xff, 0xff, 0x00, 0x00,
0x00, 0xf0, 0x07, 0xf8, 0x03, 0x00, 0x00, 0xfc, 0x00, 0xc0, 0x0f, 0x00,
0x00, 0x3e, 0x00, 0x00, 0x1f, 0x00, 0x80, 0x0f, 0x00, 0x00, 0x7c, 0x00,
0xc0, 0x07, 0x00, 0x00, 0xf8, 0x00, 0xe0, 0x01, 0x00, 0x00, 0xe0, 0x01,
0xf0, 0x00, 0x00, 0x00, 0xc0, 0x03, 0x70, 0x00, 0x00, 0x00, 0x80, 0x03,
0x38, 0x7e, 0x00, 0x80, 0x1f, 0x07, 0x38, 0xff, 0x00, 0xc0, 0x3f, 0x07,
0x9c, 0xff, 0x01, 0xc0, 0x3f, 0x0e, 0x9c, 0xe7, 0x01, 0xc0, 0x39, 0x0e,
0x8e, 0xc3, 0x01, 0xc0, 0x30, 0x1c, 0x8e, 0xe3, 0x01, 0xc0, 0x31, 0x1c,
0x86, 0xf7, 0x01, 0xc0, 0x3b, 0x18, 0x87, 0xff, 0x01, 0xc0, 0x3f, 0x38,
0x07, 0xff, 0x00, 0x80, 0x3f, 0x38, 0x03, 0x7e, 0x00, 0x80, 0x1f, 0x30,
0x03, 0x00, 0x00, 0x00, 0x00, 0x30, 0x03, 0x00, 0x00, 0x00, 0x00, 0x30,
0x03, 0x00, 0x00, 0x00, 0x00, 0x30, 0x03, 0x00, 0x00, 0x00, 0x00, 0x30,
0x03, 0x00, 0x00, 0x00, 0x00, 0x30, 0x03, 0x00, 0x00, 0x00, 0x00, 0x30,
0x07, 0x00, 0x00, 0x00, 0x00, 0x38, 0x07, 0x20, 0x00, 0x00, 0x01, 0x38,
0x06, 0x70, 0x00, 0x80, 0x03, 0x18, 0x0e, 0xf0, 0x00, 0xc0, 0x01, 0x1c,
0x0e, 0xe0, 0x03, 0xf0, 0x01, 0x1c, 0x1c, 0xc0, 0x3f, 0xfc, 0x00, 0x0e,
0x1c, 0x80, 0xff, 0x7f, 0x00, 0x0e, 0x38, 0x00, 0xfc, 0x1f, 0x00, 0x07,
0x38, 0x00, 0xc0, 0x03, 0x00, 0x07, 0x70, 0x00, 0x00, 0x00, 0x80, 0x03,
0xf0, 0x00, 0x00, 0x00, 0xc0, 0x03, 0xe0, 0x01, 0x00, 0x00, 0xe0, 0x01,
0xc0, 0x07, 0x00, 0x00, 0xf8, 0x00, 0x80, 0x0f, 0x00, 0x00, 0x7c, 0x00,
0x00, 0x3e, 0x00, 0x00, 0x1f, 0x00, 0x00, 0xfc, 0x00, 0xc0, 0x0f, 0x00,
0x00, 0xf0, 0x07, 0xf8, 0x03, 0x00, 0x00, 0xc0, 0xff, 0xff, 0x00, 0x00,
0x00, 0x00, 0xfe, 0x1f, 0x00, 0x00
};
void setup()
{
// Display starten
u8g2.begin();
// Farbe weiß
u8g2.setDrawColor(1);
}
void loop()
{
// Position 0 Grad
u8g2.clearDisplay();
u8g2.setDisplayRotation(U8G2_R0);
u8g2.firstPage();
do
{
u8g2.drawXBM(40, 10, SmileyBreite, SmileyHoehe, Smiley);
}
while (u8g2.nextPage());
delay(2000);
// Position 90 Grad
u8g2.clearDisplay();
u8g2.setDisplayRotation(U8G2_R1);
u8g2.firstPage();
do
{
u8g2.drawXBM(10, 30, SmileyBreite, SmileyHoehe, Smiley);
}
while (u8g2.nextPage());
delay(2000);
// Position 180 Grad
u8g2.clearDisplay();
u8g2.setDisplayRotation(U8G2_R2);
u8g2.firstPage();
do
{
u8g2.drawXBM(40, 10, SmileyBreite, SmileyHoehe, Smiley);
}
while (u8g2.nextPage());
delay(2000);
// Position 270 Grad
u8g2.clearDisplay();
u8g2.setDisplayRotation(U8G2_R3);
u8g2.firstPage();
do
{
u8g2.drawXBM(10, 30, SmileyBreite, SmileyHoehe, Smiley);
}
while (u8g2.nextPage());
delay(2000);
}
Letzte Aktualisierung: