Das Beispielprogramm zeigt die Möglichkeiten ein TFT-Display mit Hilfe einer Bibliothek anzusteuern.
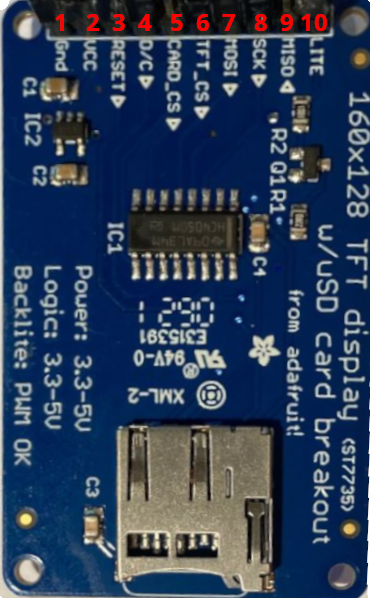
Adafruit Pinbelegung
Pin TFT | UNO R3/R4 Nano | ESP32 WROOM | Wemos D1 Mini |
---|---|---|---|
Gnd | GND | GND | GND |
VCC | 5V | 5V | 5V |
RESET | 8 | 4 | D1 |
DC | 9 | 2 | D8 |
CARD_CS | |||
TFT_CS | 10 | 5 | D0 |
SDO | 11 | 23 | D7 |
SCK | 13 | 18 | D5 |
SDI | |||
LITE | 5V | 5V | 5V |
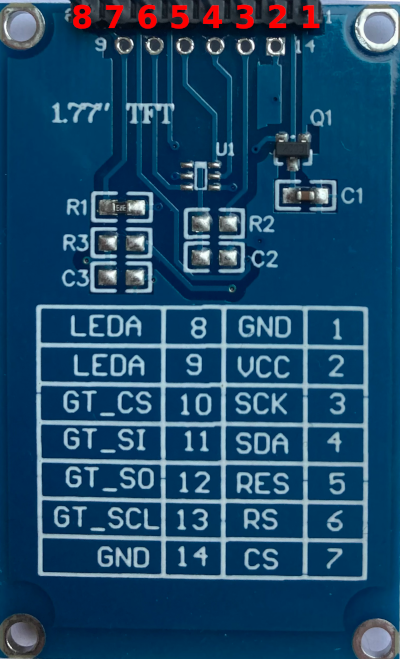
1,77 Zoll Pinbelegung
Pin TFT | UNO R3/R4 Nano | ESP32 WROOM | Wemos D1 Mini |
---|---|---|---|
GND | GND | GND | GND |
VCC | 5V | 5V | 5V |
SCK | 13 | 18 | D5 |
SDA | 11 | 23 | D7 |
RES | 8 | 4 | D1 |
RS | 9 | 2 | D8 |
CS | 10 | 5 | D0 |
LEDA | 3,3V | 3,3V | 3,3V |
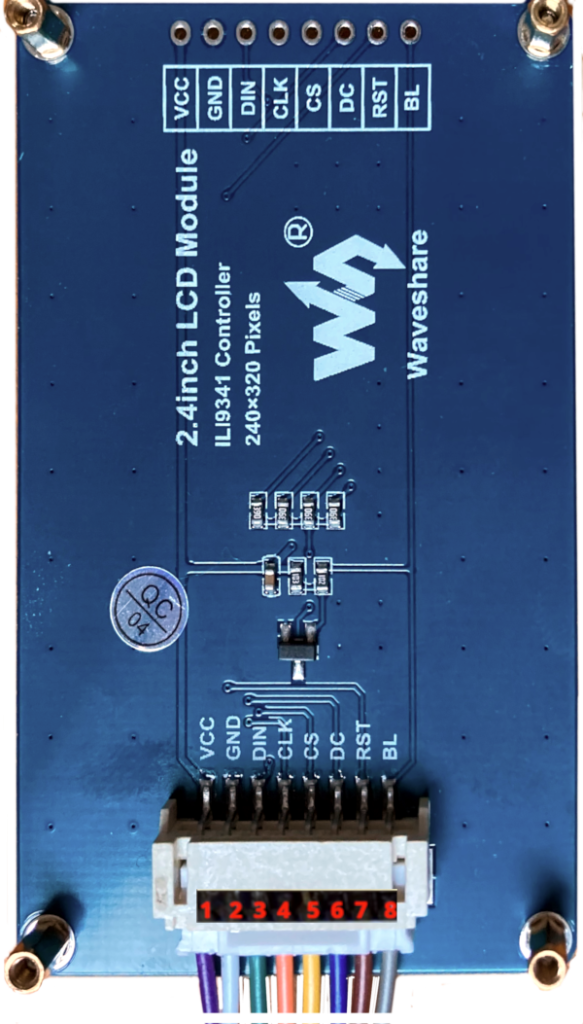
TFT Waveshare Pinbelegung
Pin TFT | UNO R3/R4 Nano | ESP32 WROOM | Wemos D1 Mini |
---|---|---|---|
VCC | 5V | 5V | 5V |
GND | GND | GND | GND |
DIN | 11 | 23 | D7 |
CLK | 13 | 18 | D5 |
CS | 10 | 5 | D0 |
DC | 9 | 2 | D8 |
RST | 8 | 4 | D1 |
BL | 5V | 5V | 5V |
Benötigte Bibliothek TFT 1,77 Zoll/1,8 Zoll:
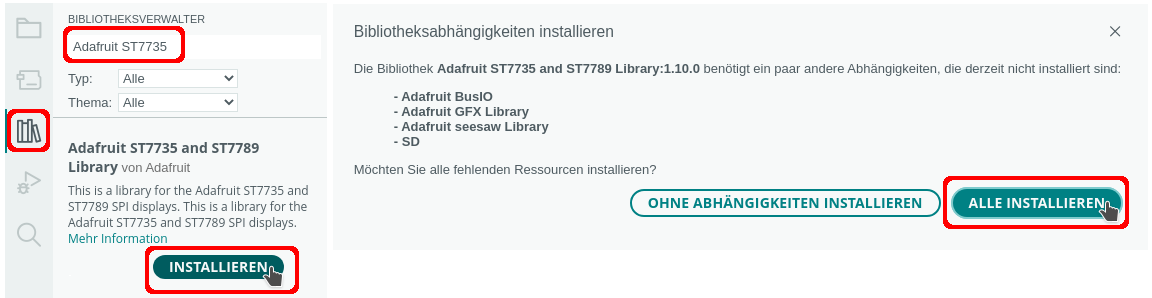
Funktionen der Bibliothek Adafruit ST7735
Schlüsselwort | Parameter | Aktion |
---|---|---|
width(); | Bildschirmbreite feststellen | |
height(); | Bildschirmhöhe feststellen | |
begin() | TFT starten | |
initR(initR(INITR_*TAB);); | BLACKTAB GREENTAB REDTAB | Farbschema bestimmen |
setRotation(Richtung); | Richtung = 0 → nicht drehen Richtung = 1 → 90° drehen Richtung = 2 → 180° drehen Richtung = 3 → 270 ° drehen | Bildschirm ausrichten |
fillScreen(Farbe); | Standardfarben: ST7735_BLACK ST7735_WHITE ST7735_GREEN ST7735_RED ST7735_BLUE ST7735_YELLOW ST7735_ORANGE ST7735_MAGENTA ST7735_CYAN | Bildschirmhintergrund |
drawLine(StartX, StartY, EndeX, EndeY, Farbe); | Linie zeichnen | |
drawFastHLine(StartX, StartY, Länge, Farbe); | horizontale Linie zeichnen | |
drawFastVLine(StartX, StartY, Länge, Farbe); | vertikale Linie zeichnen | |
drawRect(StartX, StartY,, Breite, Höhe, Farbe); | Rechteck zeichnen | |
drawRoundRect(StartX, StartY, Breite, Höhe, Eckenradius, Farbe); | abgerundetes Rechteck zeichnen | |
fillRect(StartX, StartY, Breite, Höhe, Füllfarbe); | ausgefülltes Rechteck zeichnen | |
drawCircle(MittelpunkX, MittelpunktY, Radius, Farbe); | Kreis zeichnen | |
fillCircle(MittelpunktX, MittelpunktY, Radius, Füllfarbe); | Ausgefüllten Kreis zeichnen | |
setCursor(x, y); | Cursor setzen | |
setTextSize(Textgröße); | Textgröße: 1 - 4 | Textgröße bestimmen |
setTextColor(Farbe); | Textfarbe setzen | |
print("Text"); println("Text"); | Text schreiben | |
setTextWrap(true/false); | false → Text fließt über den Rand des TFTs hinaus true → Text wird am Ende umgebrochen | Zeilenumbruch |

Beachte die unterschiedliche Definition der Variablen und den Aufruf der Bibliothek Adafruit!
Programm für den Arduino UNO
// Bibliotheken einbinden
# include "Adafruit_GFX.h"
# include "Adafruit_ST7735.h"
/*
1,77 Zoll TFT
# define TFT_PIN_CS 10
# define TFT_PIN_DC 9
# define TFT_PIN_RST 8
Adafruit_ST7735 tft = Adafruit_ST7735(TFT_PIN_CS, TFT_PIN_DC, TFT_PIN_RST);
*/
// Adafruit TFT, WaveShare TFT 1,8 Zoll
# define TFT_CS 10
# define TFT_RST 9
# define TFT_DC 8
Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST);
void setup()
{
Serial.begin(9600);
delay(500);
Serial.println("Bildschirm: " + String(tft.height()) + " x " + String(tft.width()));
tft.initR(INITR_BLACKTAB);
// Rotation anpassen
tft.setRotation(2);
// schwarzer Hintergrund
tft.fillScreen(ST7735_BLACK);
// verschiedene Schriftgrößen
tft.setTextSize(1);
tft.setCursor(1, 5);
tft.setTextColor(ST7735_BLUE);
tft.print("Text");
delay(500);
tft.setTextSize(2);
tft.setCursor(1, 20);
tft.setTextColor(ST7735_GREEN);
tft.print("Text");
delay(500);
tft.setTextSize(3);
tft.setCursor(1, 40);
tft.setTextColor(ST7735_RED);
tft.print("Text");
delay(500);
tft.setTextSize(4);
tft.setCursor(1, 70);
tft.setTextColor(ST7735_YELLOW);
tft.print("Text");
delay(2000);
// Linien ziehen
tft.fillScreen(ST7735_BLACK);
for (int i = 1; i < tft.height(); i+=10)
{
tft.drawLine(1, i, tft.width(), i, ST7735_ORANGE);
}
delay(2000);
// Kreise zeichnen
tft.fillScreen(ST7735_BLACK);
tft.fillCircle(tft.width() / 2, tft.height() / 2, 50, ST7735_MAGENTA);
tft.fillCircle(tft.width() / 2, tft.height() / 2, 30, ST7735_GREEN);
tft.fillCircle(tft.width() / 2, tft.height() / 2, 10, ST7735_YELLOW);
delay(2000);
// Rechtecke zeichnen
tft.fillScreen(ST7735_BLACK);
tft.drawRect(1, 1, 50, 50, ST7735_ORANGE);
tft.drawRect(5, 5, 50, 50, ST7735_ORANGE);
tft.drawRect(10, 10, 50, 50, ST7735_ORANGE);
delay(2000);
// ausgefüllte Rechtecke zeichnen
tft.fillScreen(ST7735_BLACK);
tft.fillRect(5, 5, 50, 50, ST7735_GREEN);
tft.fillRect(10, 10, 70, 70, ST7735_BLUE);
tft.fillRect(15, 15, 90, 90, ST7735_RED);
}
void loop()
{
// nichts zu tun, das Programm
// läuft nur einmal
}
Benötigte Bibliotheken Waveshare 2,4 Zoll
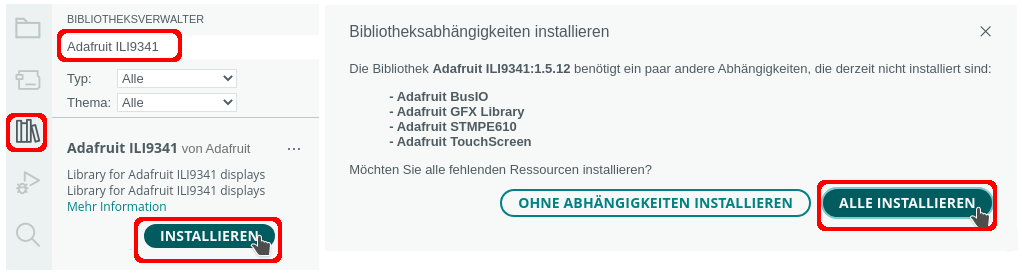
Funktionen der Bibliothek Adafruit ILI9341
Schlüsselwort | Parameter | Aktion |
---|---|---|
begin() | TFT starten | |
setRotation(Richtung); | Richtung = 0 → nicht drehen Richtung = 1 → 90° drehen Richtung = 2 → 180° drehen Richtung = 3 → 270 ° drehen | Bildschirm ausrichten |
fillScreen(Farbe); | Standardfarben: ILI9341_BLACK ILI9341_WHITE ILI9341_GREEN ILI9341_DARKGREEN ILI9341_RED ILI9341_BLUE ILI9341_NAVY ILI9341_CASET ILI9341_YELLOW ILI9341_ORANGE ILI9341_MAGENTA ILI9341_CYAN ILI9341_DARKCYAN ILI9341_LIGHTGREY ILI9341_DARKGREY ILI9341_GREENYELLOW ILI9341_MAROON | Bildschirmhintergrund |
drawLine(StartX, StartY, EndeX, EndeY, Farbe); | Linie zeichnen | |
drawFastHLine(StartX, StartY, Länge, Farbe); | horizontale Linie zeichnen | |
drawFastVLine(StartX, StartY, Länge, Farbe); | vertikale Linie zeichnen | |
drawRect(StartX, StartY,, Breite, Höhe, Farbe); | Rechteck zeichnen | |
drawRoundRect(StartX, StartY, Breite, Höhe, Eckenradius, Farbe); | abgerundetes Rechteck zeichnen | |
fill.Rect(StartX, StartY, Breite, Höhe, Füllfarbe); | ausgefülltes Rechteck zeichnen | |
drawCircle(MittelpunkX, MittelpunktY, Radius, Farbe); | Kreis zeichnen | |
fillCircle(MittelpunktX, MittelpunktY, Radius, Füllfarbe); | Ausgefüllten Kreis zeichnen | |
setCursor(x, y); | Cursor setzen | |
setTextSize(Textgröße); | Textgröße: | Textgröße setzen |
setTextColor(Farbe); | Textfarbe bestimmen | |
color565(rot, grün, blau); | Farbe als RGB setzen | |
print("Text"); println("Text"); | Text schreiben | |
setTextWrap(true/false); | false → Text fließt über den Rand des TFTs hinaus true → Text wird am Ende umgebrochen | Zeilenumbruch |
So sieht es aus:
Programm für den Arduino UNO
# include "Adafruit_GFX.h"
# include "Adafruit_ILI9341.h"
// SPI-Pins
# define TFT_CS 10
# define TFT_RST 9
# define TFT_DC 8
Adafruit_ILI9341 tft = Adafruit_ILI9341(TFT_CS, TFT_DC, TFT_RST);
// Farben RGB
int ROT = 255, GRUEN = 255, BLAU = 255;
void setup()
{
// Zufallsgenerator starten
randomSeed(analogRead(A0));
Serial.begin(9600);
delay(500);
Serial.println("Bildschirm: " + String(tft.height()) + " x " + String(tft.width()));
// TFT starten
tft.begin();
// Rotation anpassen
tft.setRotation(2);
// schwarzer Hintergrund
tft.fillScreen(ILI9341_BLACK);
tft.setTextSize(1);
tft.setCursor(1, 5);
tft.setTextColor(ILI9341_BLUE);
tft.print("Text");
delay(500);
tft.setTextSize(3);
tft.setCursor(1, 40);
tft.setTextColor(ILI9341_GREEN);
tft.print("Text");
delay(500);
tft.setTextSize(5);
tft.setCursor(1, 70);
tft.setTextColor(ILI9341_RED);
tft.print("Text");
delay(500);
tft.setTextSize(7);
tft.setCursor(1, 120);
tft.setTextColor(ILI9341_YELLOW);
tft.print("Text");
tft.setTextSize(9);
tft.setCursor(1, 200);
tft.setTextColor(ILI9341_LIGHTGREY);
tft.print("Text");
delay(2000);
// zufällige Pixel
tft.fillScreen(ILI9341_BLACK);
for (int i = 0; i < 700; i ++)
{
int PixelX = random(1, tft.width());
int PixelY = random(1, tft.height());
tft.drawPixel(PixelX, PixelY, tft.color565(random(ROT),random(GRUEN),random(BLAU)));
delay(5);
}
delay(2000);
// Linien ziehen
tft.fillScreen(ILI9341_BLACK);
for (int i = 1; i < tft.height(); i+=10)
{
tft.drawLine(1, i, tft.width(), i, ILI9341_ORANGE);
}
delay(2000);
// Kreise vom Mittelpunkt zeichnen
tft.fillScreen(ILI9341_BLACK);
for (int i = 1; i < tft.width() / 2; i+=10)
{
tft.fillCircle(tft.width() / 2, tft.height() / 2, tft.width() / 2 - i, tft.color565(random(ROT),random(GRUEN),random(BLAU)));
delay(50);
}
delay(2000);
// Rechtecke zeichnen
tft.fillScreen(ILI9341_BLACK);
for (int i = 1; i < tft.width(); i+=10)
{
tft.drawRect(tft.width() / 2 - i / 2, tft.height() / 2 - i / 2 , i, i, tft.color565(random(ROT),random(GRUEN),random(BLAU)));
}
delay(2000);
// ausgefüllte Rechtecke zeichnen
tft.fillScreen(ILI9341_BLACK);
for (int i = 1; i < tft.width() / 2; i+=10)
{
tft.fillRect(i, i, i, i, tft.color565(random(ROT),random(GRUEN),random(BLAU)));
delay(50);
}
delay(2000);
// Dreiecke
tft.fillScreen(ILI9341_BLACK);
for (int i = 1; i <tft.width(); i+=10)
{
tft.fillTriangle(i, i, 100, 100, 1, tft.width(), tft.color565(random(ROT),random(GRUEN),random(BLAU)));
delay(50);
}
delay(2000);
}
void loop()
{
// nichts zu tun, das Programm
// läuft nur einmal
}

So sieht es aus:
Letzte Aktualisierung: