Anzeige nur Text
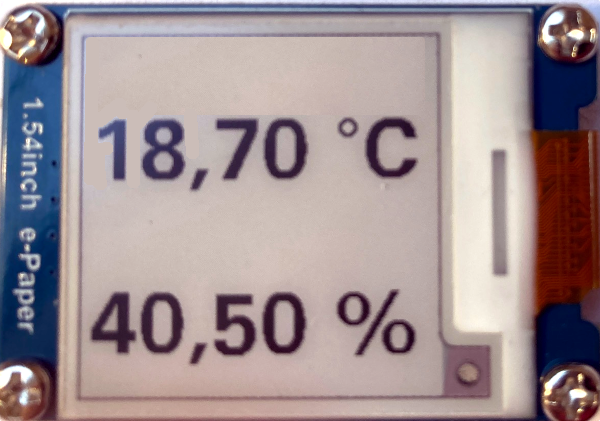
#include "GxEPD2_3C.h"
#include "DHT.h"
#include "U8g2_for_Adafruit_GFX.h"
// Objekt u8g2Schriften
U8G2_FOR_ADAFRUIT_GFX u8g2Schriften;
// freien Pin auf dem Board wählen
int SENSOR_DHT = 9;
// Sensortyp festlegen
// DHT22
#define SensorTyp DHT22
// DHT11
// #define SensorTyp DHT11
// Sensor einen Namen zuweisen
DHT dht(SENSOR_DHT, SensorTyp);
// Display-Parameter
// GxEPD2_154_Z90c: Chip SSD1681 Bildschirm: 200x200
#define GxEPD2_DISPLAY_CLASS GxEPD2_3C
#define GxEPD2_DRIVER_CLASS GxEPD2_154_Z90c
// Board wählen:
// ESP32-Wroom
// Anschlüsse: CLK -> 18, DIN -> 23, CS -> 5, DC -> 2, RST -> 22, BUSY -> 4
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ 5, /*DC=*/ 2, /*RST=*/ 22, /*BUSY=*/ 4));
// NodeMCU
// CLK - D5, DIN -> D7, CS -> D8, DC -> D6, RST -> D2, BUSY -> D1
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/D4, /*DC=*/ D6, /*RST=*/ D2, /*BUSY=*/ D1));
// ESP32-C6
// Anschlüsse: CLK -> 21, DIN -> 19, CS -> 18, DC -> 3, RST -> 10, BUSY -> 11
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ 18, /*DC=*/ 3, /*RST=*/ 2, /*BUSY=*/ 11));
// Nano ESP32
// Anschlüsse: CLK -> D13, DIN -> D11, CS -> D10, DC-> D6, RST -> D8, BUSY -> D9
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ D10, /*DC=*/ D6, /*RST=*/ D7, /*BUSY=*/ D9));
void setup()
{
Serial.begin(9600);
// auf serielle Verbindung warten
while (!Serial);
delay(1000);
// Sensor starten
dht.begin();
// Bildschirm starten
display.init(115200, true, 2, false);
// Bildschirm um 90° drehen
display.setRotation(1);
// vollständigen Bildschirm nutzen
display.setFullWindow();
// weißer Hintergrund
display.fillScreen(GxEPD_WHITE);
// Schriften von u8g2 display zuordnen
u8g2Schriften.begin(display);
}
void loop()
{
// Temperatur lesen
String Temperatur = String(dht.readTemperature());
// replace -> . durch , ersetzen
Temperatur.replace(".", ",");
// Luftfeuchtigkeit lesen
String Luftfeuchtigkeit = String(dht.readHumidity());
// replace -> . durch , ersetzen
Luftfeuchtigkeit.replace(".", ",");
// Anzeige aufbauen
display.firstPage();
do
{
display.fillScreen(GxEPD_WHITE);
u8g2Schriften.setForegroundColor(GxEPD_BLACK);
u8g2Schriften.setBackgroundColor(GxEPD_WHITE);
u8g2Schriften.setFont(u8g2_font_fub35_tf);
u8g2Schriften.setCursor(1, 80);
u8g2Schriften.print(Temperatur + " °C");
u8g2Schriften.setCursor(1, 180);
u8g2Schriften.print(Luftfeuchtigkeit + " %");
}
while (display.nextPage());
// alle 5 Minuten aktualisieren
delay(300000);
}
Anzeige nur Text mit Uhrzeit
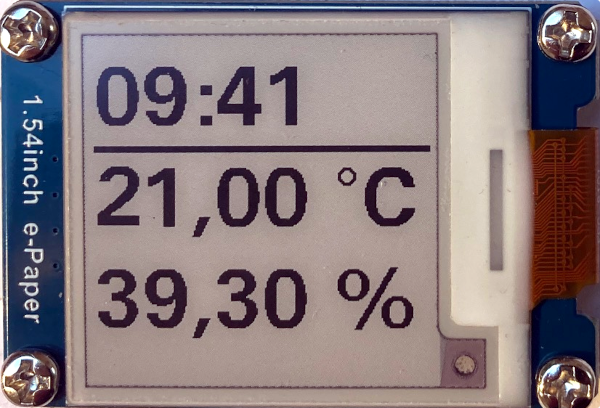
#ifdef ESP8266
#include "ESP8266WiFi.h"
#else
#include "WiFi.h"
#endif
#include "time.h"
#include "GxEPD2_3C.h"
#include "U8g2_for_Adafruit_GFX.h"
#include "DHT.h"
// Objekt u8g2Schriften
U8G2_FOR_ADAFRUIT_GFX u8g2Schriften;
// freien Pin auf dem Board wählen
int SENSOR_DHT = 9;
// Sensortyp festlegen
// DHT22
#define SensorTyp DHT22
// DHT11
// #define SensorTyp DHT11
// Sensor einen Namen zuweisen
DHT dht(SENSOR_DHT, SensorTyp);
// Schriftart
#include "Fonts/FreeMonoBold24pt7b.h"
char Router[] = "Router_SSID";
char Passwort[] = "xxxxxxxx";
// NTP-Server aus dem Pool
#define Zeitserver "de.pool.ntp.org"
/*
Liste der Zeitzonen
https://github.com/nayarsystems/posix_tz_db/blob/master/zones.csv
Zeitzone CET = Central European Time -1 -> 1 Stunde zurück
CEST = Central European Summer Time von
M3 = März, 5.0 = Sonntag 5. Woche, 02 = 2 Uhr
bis M10 = Oktober, 5.0 = Sonntag 5. Woche 03 = 3 Uhr
*/
#define Zeitzone "CET-1CEST,M3.5.0/02,M10.5.0/03"
// time_t enthält die Anzahl der Sekunden seit dem 1.1.1970 0 Uhr
time_t aktuelleZeit;
/*
Struktur tm
tm_hour -> Stunde: 0 bis 23
tm_min -> Minuten: 0 bis 59
tm_sec -> Sekunden 0 bis 59
tm_mday -> Tag 1 bis 31
tm_mon -> Monat: 0 (Januar) bis 11 (Dezember)
tm_year -> Jahre seit 1900
tm_yday -> vergangene Tage seit 1. Januar des Jahres
tm_isdst -> Wert > 0 = Sommerzeit (dst = daylight saving time)
*/
tm Zeit;
WiFiServer Server(80);
WiFiClient Client;
// Display-Parameter
// GxEPD2_154_Z90c: Chip SSD1681 Bildschirm: 200x200
#define GxEPD2_DISPLAY_CLASS GxEPD2_3C
#define GxEPD2_DRIVER_CLASS GxEPD2_154_Z90c
// Board wählen:
// ESP32-Wroom
// Anschlüsse: CLK -> 18, DIN -> 23, CS -> 5, DC -> 2, RST -> 22, BUSY -> 4
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ 5, /*DC=*/ 2, /*RST=*/ 22, /*BUSY=*/ 4));
// NodeMCU
// CLK - D5, DIN -> D7, CS -> D8, DC -> D6, RST -> D2, BUSY -> D1
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/D4, /*DC=*/ D6, /*RST=*/ D2, /*BUSY=*/ D1));
// ESP32-C6
// Anschlüsse: CLK -> 21, DIN -> 19, CS -> 18, DC -> 3, RST -> 10, BUSY -> 11
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ 18, /*DC=*/ 3, /*RST=*/ 2, /*BUSY=*/ 11));
// Nano ESP32
// Anschlüsse: CLK -> D13, DIN -> D11, CS -> D10, DC-> D6, RST -> D8, BUSY -> D9
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ D10, /*DC=*/ D6, /*RST=*/ D7, /*BUSY=*/ D9));
void setup()
{
// Schriften von u8g2 display zuordnen
u8g2Schriften.begin(display);
// Zeitzone: Parameter für die zu ermittelnde Zeit
configTzTime(Zeitzone, Zeitserver);
Serial.begin(9600);
// auf serielle Verbindung warten
while (!Serial);
delay(1000);
// WiFi starten
WiFi.begin(Router, Passwort);
Serial.println("------------------------");
while (WiFi.status() != WL_CONNECTED) {
delay(200);
Serial.print(".");
}
Serial.println();
Serial.print("Verbunden mit ");
Serial.println(Router);
Serial.print("IP über DHCP: ");
Serial.println(WiFi.localIP());
// Sensor starten
dht.begin();
// Bildschirm starten
display.init(115200, true, 2, false);
// Bildschirm um 90° drehen
display.setRotation(1);
// vollständigen Bildschirm nutzen
display.setFullWindow();
// weißer Hintergrund
display.fillScreen(GxEPD_WHITE);
}
void loop()
{
// aktuelle Zeit holen
time(&aktuelleZeit);
// localtime_r -> Zeit in die lokale Zeitzone setzen
localtime_r(&aktuelleZeit, &Zeit);
// Temperatur lesen
String Temperatur = String(dht.readTemperature());
// replace -> . durch , ersetzen
Temperatur.replace(".", ",");
// Luftfeuchtigkeit lesen
String Luftfeuchtigkeit = String(dht.readHumidity());
// replace -> . durch , ersetzen
Luftfeuchtigkeit.replace(".", ",");
// Anzeige aufbauen
display.firstPage();
do
{
display.fillScreen(GxEPD_WHITE);
u8g2Schriften.setForegroundColor(GxEPD_BLACK);
u8g2Schriften.setBackgroundColor(GxEPD_WHITE);
u8g2Schriften.setFont(u8g2_font_fub35_tf);
u8g2Schriften.setCursor(1, 50);
// Stunde: wenn Stunde < 10 -> 0 davor setzen
if (Zeit.tm_hour < 10) u8g2Schriften.print("0");
u8g2Schriften.print(Zeit.tm_hour);
u8g2Schriften.print(":");
// Minuten
if (Zeit.tm_min < 10) u8g2Schriften.print("0");
u8g2Schriften.print(Zeit.tm_min);
// horizontale Linie
display.fillRect(1, 62, display.width(), 4, GxEPD_BLACK);
u8g2Schriften.setCursor(1, 110);
u8g2Schriften.print(Temperatur + " °C");
u8g2Schriften.setCursor(1, 170);
u8g2Schriften.print(Luftfeuchtigkeit + " %");
}
while (display.nextPage());
// alle 5 Minuten aktualisieren
delay(300000);
}
Anzeige mit Symbolen und Text

#ifdef ESP8266
#include "ESP8266WiFi.h"
#else
#include "WiFi.h"
#endif
#include "time.h"
#include "GxEPD2_3C.h"
#include "U8g2_for_Adafruit_GFX.h"
#include "DHT.h"
// Objekt u8g2Schriften
U8G2_FOR_ADAFRUIT_GFX u8g2Schriften;
// Pin DHT
int SENSOR_DHT = 9;
// Sensortyp festlegen
// DHT22
#define SensorTyp DHT22
// DHT11
// #define SensorTyp DHT11
// Sensor einen Namen zuweisen
DHT dht(SENSOR_DHT, SensorTyp);
char Router[] = "Router_SSID";
char Passwort[] = "xxxxxxxx";
// NTP-Server aus dem Pool
#define Zeitserver "de.pool.ntp.org"
/*
Liste der Zeitzonen
https://github.com/nayarsystems/posix_tz_db/blob/master/zones.csv
Zeitzone CET = Central European Time -1 -> 1 Stunde zurück
CEST = Central European Summer Time von
M3 = März, 5.0 = Sonntag 5. Woche, 02 = 2 Uhr
bis M10 = Oktober, 5.0 = Sonntag 5. Woche 03 = 3 Uhr
*/
#define Zeitzone "CET-1CEST,M3.5.0/02,M10.5.0/03"
// time_t enthält die Anzahl der Sekunden seit dem 1.1.1970 0 Uhr
time_t aktuelleZeit;
/*
Struktur tm
tm_hour -> Stunde: 0 bis 23
tm_min -> Minuten: 0 bis 59
tm_sec -> Sekunden 0 bis 59
tm_mday -> Tag 1 bis 31
tm_mon -> Monat: 0 (Januar) bis 11 (Dezember)
tm_year -> Jahre seit 1900
tm_yday -> vergangene Tage seit 1. Januar des Jahres
tm_isdst -> Wert > 0 = Sommerzeit (dst = daylight saving time)
*/
tm Zeit;
WiFiServer Server(80);
WiFiClient Client;
// Display-Parameter
// GxEPD2_154_Z90c: Chip SSD1681 Bildschirm: 200x200
#define GxEPD2_DISPLAY_CLASS GxEPD2_3C
#define GxEPD2_DRIVER_CLASS GxEPD2_154_Z90c
// ESP32-Wroom
// Anschlüsse: CLK -> 18, DIN -> 23, CS -> 5, DC-> 2, RST -> 22, BUSY -> 4
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/5, /*DC=*/2, /*RST=*/22, /*BUSY=*/4));
// NodeMCU
// CLK - D5, DIN -> D7, CS -> D8, DC -> D6, RST -> D2, BUSY -> D1
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/D4, /*DC=*/D6, /*RST=*/D2, /*BUSY=*/D1));
// ESP32-C6
// Anschlüsse: CLK -> 21, DIN -> 19, CS -> 18, DC-> 3, RST -> 10, BUSY -> 11
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ 18, /*DC=*/ 3, /*RST=*/2, /*BUSY=*/11));
// Nano ESP32
// Anschlüsse: CLK -> D13, DIN -> D11, CS -> D10, DC-> D6, RST -> D8, BUSY -> D9
// GxEPD2_DISPLAY_CLASS<GxEPD2_DRIVER_CLASS, 200>
// display(GxEPD2_DRIVER_CLASS(/*CS=*/ D10, /*DC=*/ D6, /*RST=*/ D7, /*BUSY=*/ D9));
const unsigned char Thermometer [] PROGMEM = {
// 'Thermometer, 34x70px
0x00, 0x07, 0xf0, 0x00, 0x00, 0x00, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0x3f, 0xfe, 0x00, 0x00, 0x00,
0x7c, 0x0f, 0x00, 0x00, 0x00, 0x70, 0x07, 0x80, 0x00, 0x00, 0xe0, 0x03, 0xc0, 0x00, 0x00, 0xe0,
0x01, 0xc0, 0x00, 0x01, 0xc0, 0x01, 0xc0, 0x00, 0x01, 0xc0, 0x01, 0xc0, 0x00, 0x01, 0xc0, 0x01,
0xc0, 0x00, 0x01, 0xc0, 0x01, 0xc0, 0x00, 0x0f, 0xc0, 0x01, 0xc0, 0x00, 0x0f, 0xc0, 0x01, 0xc0,
0x00, 0x01, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc0, 0x01, 0xc0, 0x00,
0x00, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc0, 0x01, 0xc0, 0x00, 0x0f, 0xc0, 0x01, 0xc0, 0x00, 0x0f,
0xc0, 0x01, 0xc0, 0x00, 0x01, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc0,
0x01, 0xc0, 0x00, 0x00, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc0, 0x01, 0xc0, 0x00, 0x0f, 0xc0, 0x01,
0xc0, 0x00, 0x0f, 0xc0, 0x01, 0xc0, 0x00, 0x01, 0xc0, 0x01, 0xc0, 0x00, 0x00, 0xc3, 0xe1, 0xc0,
0x00, 0x00, 0xc3, 0xf1, 0xc0, 0x00, 0x00, 0xc3, 0xf1, 0xc0, 0x00, 0x0f, 0xc3, 0xf1, 0xc0, 0x00,
0x0f, 0xc3, 0xf1, 0xc0, 0x00, 0x0f, 0xc3, 0xf1, 0xc0, 0x00, 0x00, 0xc3, 0xf1, 0xc0, 0x00, 0x00,
0xc3, 0xf1, 0xc0, 0x00, 0x00, 0xc3, 0xf1, 0xc0, 0x00, 0x00, 0xc3, 0xf1, 0xc0, 0x00, 0x00, 0xc3,
0xf1, 0xc0, 0x00, 0x00, 0xc3, 0xf1, 0xc0, 0x00, 0x03, 0xc3, 0xf1, 0xf0, 0x00, 0x07, 0xc3, 0xf0,
0xf8, 0x00, 0x0f, 0x03, 0xf0, 0x7c, 0x00, 0x0e, 0x03, 0xe0, 0x3c, 0x00, 0x1c, 0x07, 0xf0, 0x1e,
0x00, 0x3c, 0x1f, 0xfc, 0x0f, 0x00, 0x38, 0x3f, 0xfe, 0x0f, 0x00, 0x78, 0x7f, 0xff, 0x07, 0x80,
0x70, 0x7f, 0xff, 0x87, 0x80, 0x70, 0x7f, 0xff, 0x83, 0x80, 0xf0, 0xff, 0xff, 0x83, 0xc0, 0xf0,
0xff, 0xff, 0xc3, 0xc0, 0xf0, 0xff, 0xff, 0xc3, 0xc0, 0xf0, 0xff, 0xff, 0xc3, 0xc0, 0xf0, 0xff,
0xff, 0xc3, 0xc0, 0xf0, 0xff, 0xff, 0x83, 0xc0, 0x70, 0x7f, 0xff, 0x83, 0x80, 0x70, 0x7f, 0xff,
0x87, 0x80, 0x78, 0x3f, 0xff, 0x07, 0x80, 0x38, 0x3f, 0xfe, 0x0f, 0x00, 0x3c, 0x0f, 0xfc, 0x0f,
0x00, 0x1e, 0x03, 0xe0, 0x1e, 0x00, 0x1f, 0x00, 0x00, 0x3e, 0x00, 0x0f, 0x00, 0x00, 0x7c, 0x00,
0x07, 0xe0, 0x01, 0xf8, 0x00, 0x03, 0xf8, 0x07, 0xf0, 0x00, 0x00, 0xff, 0xff, 0xc0, 0x00, 0x00,
0x7f, 0xff, 0x80, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x00, 0x00
};
const unsigned char Regen [] PROGMEM = {
// 'Regen, 60x49px
0x00, 0x00, 0x00, 0x08, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x02, 0x08, 0x21, 0x04, 0x10, 0x00, 0x00, 0x00, 0x06, 0x18, 0x63, 0x0c, 0x30, 0x80, 0x00,
0x00, 0x06, 0x10, 0x63, 0x0c, 0x31, 0x80, 0x00, 0x00, 0x00, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x20, 0x84, 0x10, 0x41, 0x08, 0x20, 0x00, 0x00, 0x61, 0x8c, 0x30, 0xc3, 0x18, 0x60, 0x00,
0x08, 0xc1, 0x8c, 0x30, 0xc3, 0x18, 0x61, 0x00, 0x00, 0x41, 0x04, 0x20, 0x82, 0x08, 0x40, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x08, 0x30, 0x86, 0x18, 0x43, 0x04, 0x30, 0x80,
0x18, 0x71, 0x86, 0x18, 0xc3, 0x0c, 0x31, 0x80, 0x18, 0x21, 0x84, 0x10, 0xc3, 0x0c, 0x21, 0x80,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x82, 0x18, 0x21, 0x04, 0x10, 0x42, 0x18, 0x40,
0x86, 0x18, 0x63, 0x0c, 0x30, 0xc6, 0x18, 0xc0, 0x86, 0x10, 0x63, 0x0c, 0x30, 0xc6, 0x18, 0xc0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x18, 0x21, 0x04, 0x10, 0xc2, 0x0c, 0x21, 0x00, 0x30, 0x63, 0x0c, 0x31, 0xc6, 0x0c, 0x63, 0x00,
0x30, 0x63, 0x0c, 0x31, 0x86, 0x18, 0x63, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x06, 0x10, 0x43, 0x08, 0x20, 0x86, 0x10, 0x00, 0x06, 0x30, 0xc3, 0x18, 0x61, 0x86, 0x30, 0x00,
0x04, 0x30, 0xc2, 0x18, 0x61, 0x8c, 0x30, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x80, 0x00, 0x00, 0x0c, 0x30, 0xc6, 0x18, 0x61, 0x00, 0x00,
0x00, 0x00, 0x61, 0xc6, 0x18, 0x60, 0x00, 0x00, 0x00, 0x00, 0x60, 0x86, 0x10, 0x60, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char Uhr [] PROGMEM = {
// Uhr, 50x50px
0x00, 0x00, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x03,
0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x1f, 0xe0, 0x01,
0xfe, 0x00, 0x00, 0x00, 0x7f, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0xfe, 0x00, 0x00, 0x1f, 0xc0,
0x00, 0x01, 0xf8, 0x00, 0x00, 0x07, 0xe0, 0x00, 0x03, 0xf0, 0x00, 0xc0, 0x03, 0xf0, 0x00, 0x07,
0xc0, 0x00, 0x00, 0x00, 0xf8, 0x00, 0x07, 0x80, 0xc0, 0x00, 0x40, 0x78, 0x00, 0x0f, 0x80, 0x00,
0x00, 0x00, 0x7c, 0x00, 0x1f, 0x00, 0x00, 0x80, 0x00, 0x3e, 0x00, 0x1e, 0x00, 0x00, 0xc0, 0x00,
0x1e, 0x00, 0x3e, 0x00, 0x00, 0xc0, 0x00, 0x1f, 0x00, 0x3c, 0x00, 0x00, 0xc0, 0x00, 0x0f, 0x00,
0x38, 0x30, 0x00, 0xc0, 0x01, 0x07, 0x00, 0x78, 0x00, 0x00, 0xc0, 0x00, 0x07, 0x80, 0x78, 0x00,
0x00, 0xc0, 0x00, 0x07, 0x80, 0x70, 0x00, 0x00, 0xc0, 0x00, 0x03, 0x80, 0xf0, 0x00, 0x00, 0xc0,
0x00, 0x03, 0xc0, 0xf0, 0x00, 0x00, 0xc0, 0x00, 0x03, 0xc0, 0xf0, 0x00, 0x00, 0xc0, 0x00, 0x03,
0xc0, 0xf0, 0x00, 0x00, 0xc0, 0x00, 0x03, 0xc0, 0xf1, 0x80, 0x00, 0xc0, 0x00, 0x63, 0xc0, 0xf1,
0x80, 0x01, 0x80, 0x00, 0x63, 0xc0, 0xf0, 0x00, 0x07, 0x00, 0x00, 0x03, 0xc0, 0xf0, 0x00, 0x1c,
0x00, 0x00, 0x03, 0xc0, 0xf0, 0x00, 0x78, 0x00, 0x00, 0x03, 0xc0, 0xf0, 0x00, 0xe0, 0x00, 0x00,
0x03, 0xc0, 0x70, 0x03, 0x80, 0x00, 0x00, 0x03, 0x80, 0x78, 0x07, 0x00, 0x00, 0x00, 0x07, 0x80,
0x78, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80, 0x78, 0x20, 0x00, 0x00, 0x01, 0x07, 0x80, 0x3c, 0x00,
0x00, 0x00, 0x00, 0x0f, 0x00, 0x3e, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x1e, 0x00, 0x00, 0x00,
0x00, 0x1e, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x3e, 0x00, 0x0f, 0x80, 0x00, 0x00, 0x00, 0x7c,
0x00, 0x07, 0x80, 0x80, 0x00, 0xc0, 0x78, 0x00, 0x07, 0xc0, 0x00, 0x00, 0x00, 0xf8, 0x00, 0x03,
0xf0, 0x00, 0xc0, 0x03, 0xf0, 0x00, 0x01, 0xf8, 0x00, 0xc0, 0x07, 0xe0, 0x00, 0x00, 0xfe, 0x00,
0x00, 0x1f, 0xc0, 0x00, 0x00, 0x7f, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x1f, 0xe0, 0x01, 0xfe,
0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xf0, 0x00, 0x00,
0x00, 0x00, 0x7f, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xfc, 0x00, 0x00, 0x00
};
void setup()
{
// Schriften von u8g2 display zuordnen
u8g2Schriften.begin(display);
// Zeitzone: Parameter für die zu ermittelnde Zeit
configTzTime(Zeitzone, Zeitserver);
Serial.begin(9600);
// auf serielle Verbindung warten
while (!Serial);
delay(1000);
// WiFi starten
WiFi.begin(Router, Passwort);
Serial.println("------------------------");
while (WiFi.status() != WL_CONNECTED) {
delay(200);
Serial.print(".");
}
Serial.println();
Serial.print("Verbunden mit ");
Serial.println(Router);
Serial.print("IP über DHCP: ");
Serial.println(WiFi.localIP());
// Bildschirm starten
display.init(115200, true, 2, false);
// Bildschirm um 90° drehen
display.setRotation(1);
// vollständigen Bildschirm nutzen
display.setFullWindow();
display.fillScreen(GxEPD_WHITE);
// Sensor starten
dht.begin();
}
void loop()
{
// aktuelle Zeit holen
time(&aktuelleZeit);
// localtime_r -> Zeit in die lokale Zeitzone setzen
localtime_r(&aktuelleZeit, &Zeit);
// Temperatur lesen
String Temperatur = String(dht.readTemperature());
// replace -> . durch , ersetzen
Temperatur.replace(".", ",");
// Luftfeuchtigkeit lesen
String Luftfeuchtigkeit = String(dht.readHumidity());
// replace -> . durch , ersetzen
Luftfeuchtigkeit.replace(".", ",");
// Anzeige aufbauen
display.firstPage();
do
{
u8g2Schriften.setForegroundColor(GxEPD_BLACK);
u8g2Schriften.setBackgroundColor(GxEPD_WHITE);
u8g2Schriften.setFont(u8g2_font_helvB24_tf);
u8g2Schriften.setCursor(70, 40);
// Stunde: wenn Stunde < 10 -> 0 davor setzen
if (Zeit.tm_hour < 10) u8g2Schriften.print("0");
u8g2Schriften.print(Zeit.tm_hour);
u8g2Schriften.print(":");
// Minuten
if (Zeit.tm_min < 10) u8g2Schriften.print("0");
u8g2Schriften.print(Zeit.tm_min);
// x, y, Bild-Array, Breite, Höhe, Farbe
display.drawBitmap(5, 5, Uhr, 50, 50, GxEPD_BLACK);
display.drawBitmap(15, 70, Thermometer, 34, 70, GxEPD_BLACK);
display.drawBitmap(5, 150, Regen, 60, 49, GxEPD_BLACK);
// Messdaten anzeigen
u8g2Schriften.setCursor(70, 110);
u8g2Schriften.print(Temperatur + " °C");
u8g2Schriften.setCursor(70, 180);
u8g2Schriften.print(Luftfeuchtigkeit + " %");
}
while (display.nextPage());
// alle 5 Minuten aktualisieren
delay(300000);
}
Letzte Aktualisierung: